If you're new to Firebase, you'll need to
create your account and install some tools first. Install latest version of node.js first
1. Create a Firebase account at https://firebase.google.com/console/.
Once your account has been created and you've signed in, you're ready to deploy!
2. Create a new Firebase app at https://firebase.google.com/console/
3. Install the Firebase tools via npm (run this in your command line).The Firebase Command Line
Interface (CLI) will allow you to serve the web app locally and deploy.
npm -g install firebase-tools
4. To verify that the CLI has been installed correctly, open a console and run:
firebase --version
5. If you haven't recently signed in to the Firebase tools, update your credentials:
firebase login
6. Make sure you are in the /app
folder (which is our project root folder i.e where our
index.html
is) then Initialize your app to use Firebase. Select your Project ID and follow
the instructions.
When prompted, you can choose any Alias, such as pwafire
for instance. Select Firebase
Hosting as the Firebase CLI feature. Provide the default directory as /
. To the other
prompts, reply NO; N
firebase init
7. Let's serve and test the firebase project locally. Run the following command from the root of your
local project directory.
firebase serve
8. Finally, deploy the app to Firebase:
firebase deploy
9. Celebrate. You're done! Your app will be deployed to the domain:
https://YOUR-FIREBASE-APP.firebaseapp.com
10. Visit your web app on your phone now. You should see an "install to homescreen" banner
prompt! Welcome to PWAs World! Further reading: Firebase Hosting Guide.
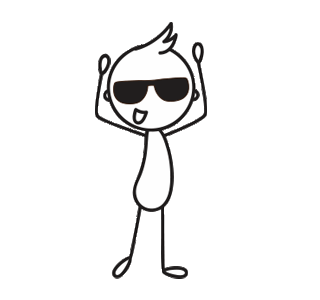